Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | 6 | |
7 | 8 | 9 | 10 | 11 | 12 | 13 |
14 | 15 | 16 | 17 | 18 | 19 | 20 |
21 | 22 | 23 | 24 | 25 | 26 | 27 |
28 | 29 | 30 | 31 |
Tags
- python수업
- 파이썬시각화
- 주피터노트북그래프
- 데이터분석시각화
- 주피터노트북판다스
- 맷플롯립
- SQL수업
- python알고리즘
- 수업기록
- SQL
- 판다스그래프
- python데이터분석
- 파이썬차트
- sql연습
- matplotlib
- sql연습하기
- 판다스데이터분석
- 파이썬수업
- Python
- 파이썬알고리즘
- sql따라하기
- 주피터노트북맷플롯립
- 파이썬데이터분석주피터노트북
- 주피터노트북
- 주피터노트북데이터분석
- 팀플기록
- 파이썬크롤링
- SQLSCOTT
- 파이썬데이터분석
- 파이썬
Archives
- Today
- Total
IT_developers
Python 개념 및 실습 - 함수(1) 본문
function
- 반복적으로 수행되는 부분을 함수록 작성
- 단독 실행 가능
- 타입을 쓰지 않음
- 변수명만 잘 써주면 됨
- def 함수명 () :
- 수행할 문장1
- 수행할 문장2
# 함수 작성
def hello():
print("hello!!!")
# 함수 사용
hello()
def hello2():
return "hello!!!"
print(hello2())
def add(a, b):
return a + b
result = add(3, 4)
print(result)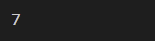
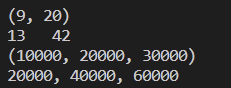
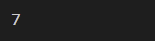
return : ,를 기준으로 리턴값을 여러개 줄수 있음. 다중 리턴은 아님.
def sum_and_mul(a, b):
return a + b, a * b # 여러개로 return 하지 않고 tuple을 사용해서 리턴됨.
print(sum_and_mul(4, 5))
# 값을 따로 받을 수 있음
add, mul = sum_and_mul(7, 6)
print(add, " ", mul)
def func_mul(x):
y1 = x * 100
y2 = x * 200
y3 = x * 300
return y1, y2, y3
print(func_mul(100))
y1, y2, y3 = func_mul(200)
print("%d, %d, %d" % (y1, y2, y3))
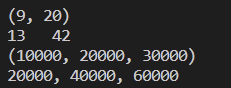
# 기본 파라메터
def print_n_times(value, n=2): # n값이 없으면 기본으로 두번 돌려줘
for i in range(n):
print(value)
print_n_times("안녕하세요")
print()
print_n_times("안녕하세요", 5)
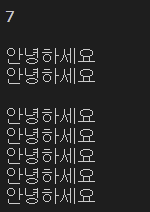
def say_myself(name, old, man=True): # 초기화시키고 싶은 입력 변수는 뒤쪽에 선언
print("나의 이름은 %s 입니다" % name)
print("나의 이름은 %d 입니다" % old)
if man:
print("남자입니다.")
else:
print("여자입니다.")
say_myself("홍길동", 27)
say_myself("홍길동", 27, False)
# *args : 가변 파라메터(인자의 개수가 정확하지 않을 때)
def add_many(*args):
print(type(args))
print(args)
add_many({"dessert": "macaroon", "wine": "merlot"})
add_many("35", "24", "15", "36")
add_many([35, 24, 18, 17])
add_many(1, 2, 3, 4, 5, 6)
add_many()

def add_many(*args): # * : 가변, tuple임
result = 0
for i in args:
result += i
return result
print("result = ", add_many())
print("result = ", add_many(15, 63, 45, 356, 36, 9, 3))
print("result = ", add_many(27, 35, 56))
print("result = ", add_many(39, 48, 17, 16, 15))
# **kwargs: 가변 파라메터를 딕셔너리 형태로 처리
def args_func1(**kwargs):
print("kwargs ", kwargs)
for k in kwargs.keys():
print(k)
for k, v in kwargs.items():
print(k, v)

args_func1(name="Kim")
args_func1(name="Kim", name2="Park", active="Test")
args_func1(name="Kim", age=10, title="Title")
args_func1(name="Kim", age=25, addr="서울")
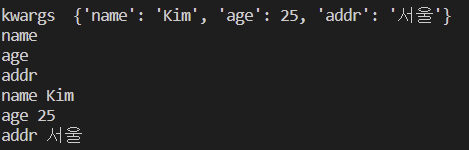
# 실습 : 같이 사용 할 수 있음
def example_mul(arg1, arg2=5, *args, **kwargs):
print(arg1, arg2, args, kwargs)
example_mul(10, 20)
example_mul(10)
example_mul(10, 20, "park", "Kim", 10, 20)
example_mul(10, 20, "choi", age=25, name="Kim")
# 함수 안에서 선언된 변수의 효력 범위
a = 1
def var_test(a):
a = a + 1
var_test(a)
print(a)
# 변경된 값 출력
a = 1
def var_test(a):
a = a + 1
return a
# var_test(a)
a = var_test(a)
print(a)

# global : 함수 밖에 있는 값을 불러서 씀. global보단 return 타입을 선호
def var_test():
global a
a = a + 1
var_test()
print(a)
실습 : 사칙 연산을 수행하는 함수(four_rules) 작성
# 인자 2개와 연산자는 사용자에게 입력받아 사칙 연산 수행
def four_rules(num1, num2, op):
if op == "+":
return num1 + num2
elif op == "-":
return num1 - num2
elif op == "*":
return num1 * num2
else:
return num1 / num2
num1 = int(input("Num1 : "))
num2 = int(input("Num2 : "))
op = input("+, -, *, / 중 하나의 연산자 입력 : ")
print("%d %c %d = %d" % (num1, op, num2, four_rules(num1, num2, op)))
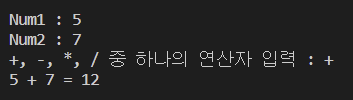
# [[1,2,3,],[4,5,6],[7,8,9]] 형태의 리스트를 받아 1차원 리스트로 반환 [1,2,3,4,5,6,7,8,9]
def flatten(data):
output = []
for item in data:
if type(item) == list:
output += flatten(item)
else:
output.append(item)
return output
list1 = [[1,2,3,],[4,5,6],[7,8,9]]
print(flatten(list1))
# 이중 for문도 가능
# 로또 생성기
import random
def get_number():
return random.randrange(1, 46)
# lotto 리스트에 6개이 숫자가 다 채워질때까지 get_number()를 호출하는 코드 작성
lotto = []
while True:
num = get_number()
# 중복제거
if lotto.count(num) == 0:
lotto.append(num)
# 무한루프
if len(lotto) >= 6:
break
lotto.sort()
print("이번 주 로또 번호 : ", lotto)
'Python' 카테고리의 다른 글
Python 개념 및 실습 - 파일 읽고 쓰기(1) (0) | 2022.09.09 |
---|---|
Python 개념 및 실습 - 함수(2) (0) | 2022.09.08 |
Python 개념 및 실습 - set (0) | 2022.09.07 |
Python 개념 및 실습 - dictionary (0) | 2022.09.07 |
Python 개념 및 실습 - tuple (0) | 2022.09.06 |
Comments