Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | 6 | |
7 | 8 | 9 | 10 | 11 | 12 | 13 |
14 | 15 | 16 | 17 | 18 | 19 | 20 |
21 | 22 | 23 | 24 | 25 | 26 | 27 |
28 | 29 | 30 | 31 |
Tags
- Python
- 주피터노트북맷플롯립
- 파이썬데이터분석
- 파이썬
- 주피터노트북판다스
- sql연습
- SQL
- 주피터노트북
- matplotlib
- 파이썬수업
- 파이썬알고리즘
- 주피터노트북데이터분석
- 판다스데이터분석
- python수업
- sql연습하기
- 수업기록
- 주피터노트북그래프
- SQL수업
- 맷플롯립
- sql따라하기
- 파이썬크롤링
- 데이터분석시각화
- 파이썬데이터분석주피터노트북
- 파이썬차트
- python알고리즘
- 팀플기록
- 판다스그래프
- 파이썬시각화
- SQLSCOTT
- python데이터분석
Archives
- Today
- Total
IT_developers
Python 개념 및 실습 - 클래스(2) 본문
# 클래스 변수와 인스턴스 변수 차이
class Car:
"""
UserInfo class
Author : 홍길동
Date : 2022-05-26
Description : 클래스 작성법
"""
# 클래스 변수
car_count = 0
# 인자를 받는 생성자
def __init__(self, count, color, speed) -> None:
self.color = color
self.speed = speed
Car.car_count += count
def upSpeed(self, value):
self.speed += value
def downSpeed(self, value):
self.speed -= value
# 객체 삭제
def __del__(self):
Car.car_count -= 1
# 객체 생성
car1 = Car(1, "Red", 20)
car1.upSpeed(50)
print(
"자동차 1 현재 속도 {}km, 색상 : {}, 생성된 자동차 대수 : {}대".format(
car1.speed, car1.color, Car.car_count
)
)
car2 = Car(1, "Black", 30)
car2.upSpeed(80)
print(
"자동차 2 현재 속도 {}km, 색상 : {}, 생성된 자동차 대수 : {}대".format(
car2.speed, car2.color, Car.car_count
)
)
car3 = Car(1, "Yellow", 0)
car3.upSpeed(100)
print(
"자동차 3 현재 속도 {}km, 색상 : {}, 생성된 자동차 대수 : {}대".format(
car3.speed, car3.color, Car.car_count
)
)
클래스 변수 : "클래스명."으로 접근 ==> Car.car_count
인스턴스 변수 : "변수명"으로 접근 ==> car3.color

# 삭제
del car1
print("생산된 자동차 대수 {}대".format(Car.car_count))
클래스 메소드
class Test:
# 인자로 self가 없는 경우 class 메소드
def function1():
print("function1 호출")
def function2(self):
print("function2 호출")
obj1 = Test()
obj1.function1() #TypeError: Test.function1() takes 0 positional arguments but 1 was given
obj1.function2() # self가 있으면 에러가 안남.
# obj1.function1과 obj1.function2의 차이는 self가 유무 여부
Test.function1() # 클래스 명으로 호출
Test.function2() # TypeError: Test.function2() missing 1 required positional argument: 'self'
self가 있다면, obj1.function2()
self가 없다면, Test.function1()
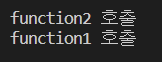
생성자 오버로딩 기능
# 파이썬은 오버로딩 기능 없음 - 초기값 이용
class UserInfo:
"""
UserInfo class
Author : 홍길동
Date : 2022-05-26
Description : 클래스 작성법
"""
user_cnt = 0
# email=None 초기값 이용
def __init__(self, name, age, email=None) -> None:
self.name = name
self.age = age
self.email = email
UserInfo.user_cnt += 1
def user_info(self):
return "name : {}, age : {}, email : {}".format(self.name, self.age, self.email)
def __del__(self):
UserInfo.user_cnt -= 1
# email=None 미입력시 오류뜸
user1 = UserInfo("홍길동", 30)
print(user1.user_info())
user2 = UserInfo("성춘향", 30, "sung@naver.com")
print(user2.user_info())
실습 : 사칙연산 가능한 클래스
class FourCal 작성 : 숫자 2개, +, -, *, / 메소드 작성(연산결과 리턴)
class FourCal:
def __init__(self, num1, num2) -> None:
self.num1 = num1
self.num2 = num2
def add(self):
return self.num1 + self.num2
def sub(self):
return self.num1 - self.num2
def mul(self):
return self.num1 * self.num2
def div(self):
return self.num1 / self.num2
num1, num2 = 10, 5
calc = FourCal(num1, num2)
print("{} + {} = {}".format(num1, num2, calc.add()))
print("{} - {} = {}".format(num1, num2, calc.sub()))
print("{} * {} = {}".format(num1, num2, calc.mul()))
print("{} / {} = {}".format(num1, num2, calc.div()))
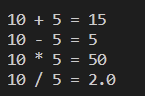
Audio 클래스
Audio 작성 : power, volume
# switch 메소드(on_off 매개 변수를 받아 power 변경)
# set_volume(volume 매개 변수를 받아 volume 변경)
# tune() : power 값이 true 일 경우 "La La La ..." else 일 경우 "turn it on"
class Audio:
def __init__(self, power, volume) -> None:
self.power = power
self.volume = volume
def switch(self, on_off):
self.power = on_off
def set_volume(self, volume):
self.volume = volume
def tune(self):
msg = "La La La ..." if self.power else "turn it on"
print(msg)
audio1 = Audio(False, 9)
audio1.switch(True)
audio1.set_volume(15)
audio1.tune()
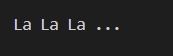
클래스 상속 : class 상속받을 클래스명(상속할 클래스명)
class Parent:
def __init__(self) -> None:
self.value = "테스트"
print("Parent 클래스의 __init__호출")
def test(self):
print("Parent 클래스의 test 호출")
class Child(Parent):
def __init__(self) -> None:
Parent.__init__(self) # 부모의 객체가 생성될 수 있도록 명시
print("Child 클래스의 __init__호출")
child = Child()
child.test()
print(child.value) # AttributeError: 'Child' object has no attribute 'value' ==> Parent.__init__(self) 추가
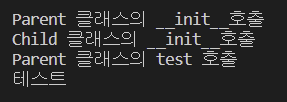
# Car 클래스 상속 ==> Sedan, Truck
class Car:
speed = 0
def up_speed(self, value):
self.speed = self.speed + value
def down_speed(self, value):
self.speed = self.speed - value
#상속받는 클래스
class Sedan(Car):
seatNum = 0
def getSeatNum(self):
return self.seatNum
#상속받는 클래스
class Truck(Car):
capacity = 0
def getCapacity(self):
return self.capacity
# 객체생성
sedan1, truck1 = Sedan(), Truck()
sedan1.up_speed(100)
truck1.up_speed(80)
sedan1.seatNum = 5
truck1.capacity = 50
print("승용차의 속도는 {}km, 좌석수는 {}개".format(sedan1.speed, sedan1.getSeatNum()))
print("트럭의 속도는 {}km, 총 중량은 {}톤".format(truck1.speed, truck1.getCapacity()))
# AudioVisual 상속 클래스 ==> Audio, TV
class AudioVisual:
def __init__(self, power, volume) -> None:
self.power = power
self.volume = volume
def switch(self, on_off):
self.power = on_off
def set_volume(self, volume):
self.volume = volume
#상속받는 클래스
class Audio(AudioVisual):
def __init__(self, power, volume):
super().__init__(power, volume)
def tune(self):
msg = "La La La ..." if self.power else "turn it on"
print(msg)
#상속받는 클래스, size 추가
class TV(AudioVisual):
def __init__(self, power, volume, size) -> None:
super().__init__(power, volume)
self.size = size
def watch(self):
msg = "have fun!!" if self.power else "switch on"
print(msg)
tv1 = TV(False, 12, 55)
tv1.switch(True)
tv1.watch()
audio1 = Audio(True, 15)
audio1.set_volume(6)
audio1.tune()
def __init__(self) -> None: return 타입이 없음을 명시
'Python' 카테고리의 다른 글
Python 개념 및 실습 - 모듈(1) (0) | 2022.09.12 |
---|---|
Python 개념 및 실습 - 클래스(3) (0) | 2022.09.11 |
Python 개념 및 실습 - 클래스(1) (1) | 2022.09.11 |
Python 개념 및 실습 - 파일 읽고 쓰기(4) (0) | 2022.09.10 |
Python 개념 및 실습 - 파일 읽고 쓰기(3) (0) | 2022.09.10 |
Comments