Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | 6 | |
7 | 8 | 9 | 10 | 11 | 12 | 13 |
14 | 15 | 16 | 17 | 18 | 19 | 20 |
21 | 22 | 23 | 24 | 25 | 26 | 27 |
28 | 29 | 30 | 31 |
Tags
- sql연습하기
- 주피터노트북판다스
- matplotlib
- SQL수업
- SQL
- 파이썬수업
- 파이썬
- 팀플기록
- python수업
- sql따라하기
- Python
- 수업기록
- 주피터노트북맷플롯립
- 주피터노트북
- SQLSCOTT
- 파이썬크롤링
- 주피터노트북데이터분석
- python알고리즘
- 파이썬차트
- 파이썬시각화
- 파이썬알고리즘
- 판다스데이터분석
- python데이터분석
- 파이썬데이터분석
- 파이썬데이터분석주피터노트북
- sql연습
- 판다스그래프
- 데이터분석시각화
- 맷플롯립
- 주피터노트북그래프
Archives
- Today
- Total
IT_developers
Python RPA(업무자동화) 개념 및 실습 - 엑셀(3) 본문
RPA(Robotic Process Automation)
- 웹, 윈도우, 어플리케이션(엑셀 등)을 사전에 설정한 시나리오에 따라 자동적으로 작동하여 수작업을 최소화하는 일련의 프로세스
- RPA 사용 소프트웨어
- Uipath, BluePrism, Automation Anywhere, WinAutomation
- RPA 라이브러리
- pyautogui, pyperclip, selenium
RPAbasic\excel 폴더 - 11_cell_style.py
셀 스타일 변경
# 라이브러리
from openpyxl import load_workbook
from openpyxl.styles import Font, Border, PatternFill, Alignment
from openpyxl.styles.borders import Side
wb = load_workbook("./RPAbasic/excel/range.xlsx")
ws = wb.active
# 셀 너비 조절 : A열
ws.column_dimensions["A"].width = 5
# 행 높이 조절 : 1행
ws.row_dimensions[1].height = 50
# 폰트 스타일
a1 = ws["A1"]
b1 = ws["B1"]
c1 = ws["C1"]
# 폰트 객체
a1.font = Font(color="ff0000", italic=True, bold=True)
b1.font = Font(color="cc33ff", name="Arial", strike=True)
c1.font = Font(color="0000ff", size=20, underline="single")
# 테두리 적용
thin_border = Border(
left=Side(style="thin"),
right=Side(style="thin"),
top=Side(style="thin"),
bottom=Side(style="thin"),
)
a1.border = thin_border
b1.border = thin_border
c1.border = thin_border
# 셀 배경색
for row in ws.rows:
for cell in row:
# 셀정렬
cell.alignment = Alignment(horizontal="center", vertical="center")
if cell.column == 1:
continue
# cell.value 가 정수형 데이터이고 값이 80보다 큰 경우
if isinstance(cell.value, int) and cell.value > 80:
cell.fill = PatternFill(fgColor="00ff00", fill_type="solid")
cell.font = Font(color="ff0000")
# 틀 고정
ws.freeze_panes = "B2"
wb.save("./RPAbasic/excel/range_style.xlsx")
RPAbasic\excel 폴더 - 12_formula.py
함수 값 삽입
from openpyxl import Workbook
from datetime import datetime
wb = Workbook()
ws = wb.active
# 날짜 넣기
ws["a1"] = datetime.today()
ws["a2"] = "=sum(1,2,3)"
ws["a3"] = "=average(1,2,3)"
ws["a4"] = 10
ws["a5"] = 20
ws["a6"] = "=sum(a4:a5)"
wb.save("./RPAbasic/excel/formula.xlsx")
# 데이터 값 가지고 오기 : 수식이 들어 있는 경우 수식 그대로 읽어옴
wb = load_workbook("./RPAbasic/excel/formula.xlsx")
for row in ws.values:
for cell in row:
print(cell)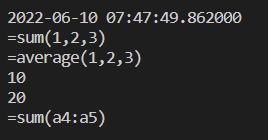
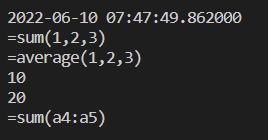
# data_only만 읽어옴. 다른 수식은 None
wb = load_workbook("./RPAbasic/excel/formula.xlsx", data_only=True)
ws = wb.active
for row in ws.values:
for cell in row:
print(cell)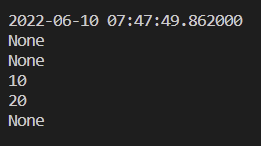
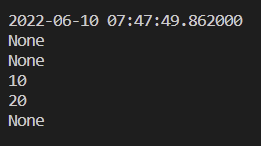
RPAbasic\excel 폴더 - 13_merge.py
셀 병합
from openpyxl import Workbook
wb = Workbook()
ws = wb.active
# 병합 셀 범위 지정
ws.merge_cells("B2:D2")
ws["B2"].value = "Merged cell"
wb.save("./RPAbasic/excel/merge.xlsx")
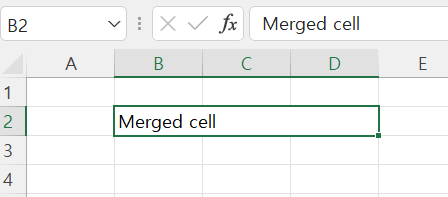
셀 병합 해지
rom openpyxl import load_workbook
wb = load_workbook("./RPAbasic/excel/merge.xlsx")
ws = wb.active
# 병합 셀 범위 지정
ws.unmerge_cells("B2:D2")
wb.save("./RPAbasic/excel/unmerge.xlsx")
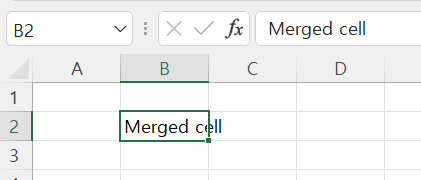
RPAbasic\excel 폴더 - 14_image.py
이미지 추가하기 : 이미지 파일을 폴더에 넣어놓기
from openpyxl import Workbook
from openpyxl.drawing.image import Image
wb = Workbook()
ws = wb.active
# ImportError: You must install Pillow to fetch image objects
# 추가 라이브러리 필요 : pip install Pillow
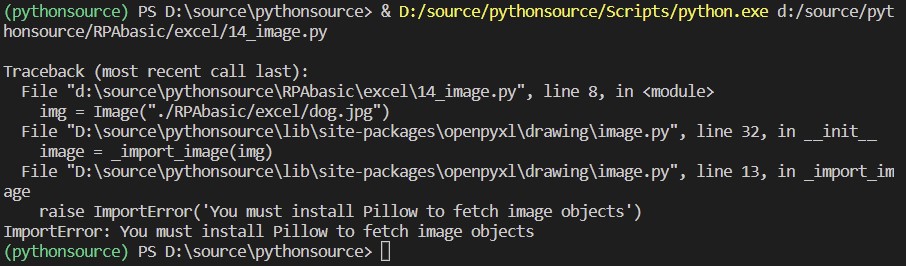

img = Image("./RPAbasic/excel/dog.jpg")
ws.add_image(img, "C3")
wb.save("./RPAbasic/excel/image.xlsx")
RPAbasic\excel 폴더 - 15_exam.py
실습 : 1번 ~ 10번 학생들 성적 관리
- 마지막 수업을 모두 마치고 최종 성적을 검토하는 과정에서 퀴즈2 문제에 오류를 발견하여 모두 만점 처리
- 데이터를 작성한 후 아래와 같이 수정
- 퀴즈2 점수를 10으로 수정
- H열에 총점(SUM 이용), I열에 성적 정보 추가
- 총점 90이상 A, 80이상 B, 70이상 C, 나머지 D
- 출석이 5미만인 학생은 총점 상관없이 F
- 최종 파일명 : scores.xlsx
학번 | 출석 | 퀴즈1 | 퀴즈2 | 중간 고사 |
기말 고사 |
프로 젝트 |
1 | 10 | 8 | 5 | 14 | 26 | 12 |
2 | 7 | 3 | 7 | 15 | 24 | 18 |
3 | 9 | 5 | 8 | 8 | 12 | 4 |
4 | 7 | 8 | 7 | 17 | 21 | 18 |
5 | 7 | 8 | 7 | 16 | 25 | 15 |
6 | 3 | 5 | 8 | 8 | 17 | 0 |
7 | 4 | 9 | 10 | 16 | 27 | 18 |
8 | 6 | 6 | 6 | 15 | 19 | 17 |
9 | 10 | 10 | 9 | 19 | 30 | 19 |
10 | 9 | 8 | 8 | 20 | 25 | 20 |
from openpyxl import Workbook
wb = Workbook()
ws = wb.active
# 타이틀 넣기
ws.append(["출석", "퀴즈1", "퀴즈2", "중간고사", "기말고사", "프로젝트", "총합"])
# 2차원
scores = [
[1, 10, 8, 5, 14, 26, 12],
[2, 7, 3, 7, 15, 24, 18],
[3, 9, 5, 8, 8, 12, 4],
[4, 7, 8, 7, 17, 21, 18],
[5, 7, 8, 7, 16, 25, 15],
[6, 3, 5, 8, 8, 17, 0],
[7, 4, 9, 10, 16, 27, 18],
[8, 6, 6, 6, 15, 19, 17],
[9, 10, 10, 9, 19, 30, 19],
[10, 9, 8, 8, 20, 25, 20],
]
for score in scores:
ws.append(score)
# 퀴즈2 점수 10점으로 변경
for idx, cell in enumerate(ws["D"]):
if idx == 0: # 제목 행 skip
continue
cell.value = 10 # 점수 value 값만 10으로 변경
# 총점, 성적 셀 추가
ws["H1"].value = "총점"
ws["I1"].value = "성적"
# 성적 계산
for idx, score in enumerate(scores, start=2):
# format을 이용해서 값을 지정.
ws.cell(row=idx, column=8).value = "=sum(B{0}:G{0})".format(idx)
# 성적
total = sum(score[1:]) - score[3] + 10
grade = None
if total >= 90:
grade = "A"
elif total >= 80:
grade = "B"
elif total >= 70:
grade = "C"
else:
grade = "D"
if score[1] < 5:
grade = "F"
ws.cell(row=idx, column=9).value = grade
wb.save("./RPAbasic/excel/scores.xlsx")
'Python' 카테고리의 다른 글
Python RPA(업무자동화) 개념 및 실습 - 크롤링(urllib) (1) | 2022.09.25 |
---|---|
Python RPA(업무자동화) 개념 및 실습 - pyautogui (1) | 2022.09.24 |
Python RPA(업무자동화) 개념 및 실습 - 엑셀(2) (1) | 2022.09.22 |
Python RPA(업무자동화) 개념 및 실습 - 엑셀(1) (1) | 2022.09.21 |
Python algorithm 개념 및 실습 - 최대 수익 (1) | 2022.09.20 |
Comments